Overview
when I started learning golang, I was really missing python where I had an option to check part of my codes immediately as needed. I Started looking for some available options but couldn't find a full-fledged interpreter for this compiled language.
So given the requirement, I decided to make my own. This is a dummy interpreter for go written in python with some limitations and there is more improvement to do here. Unless we don't find any standard similar tool, still, it enables you to check your codes snippets, goroutines, any package and many more. you should definitely give it try so that you don't get stuck with building and then testing large system. just because you have doubt in small and silly things of go constructs.
For more information on gointerpreter, you can also check the link below :
For more information of go please refer standard go website:
Why to use Gointerpreter
one can use gointerpreter to aid their golang learning Process. Users can check the behavior of golang constructs on the fly as they are learning.
Usage
To perform some administrative tasks Gointerpreter also understands some meta-commands that are not part of golang language. Meta-commands are always preceded by a ":" , examples are :x, :h, :help, :c etc. Descriptions of some commands are as below:
Metaprocessor Commands
Command | Usage |
---|---|
:h CommandName | display brief descriptions of all meta-commands. |
:load <filename> | Loads from file. |
:pp <True|False> | enable/disable prettyprinter. |
:CGO | Cgo input (use KeyboardInterrupt to save). |
:e | Edit the Source File(in editor or single line). |
:r, :x | Run as Go File (gointerpreter actually renders and executes the go file in background. |
:c | Clear the session,and Restart. |
:save <filename> | Save the current session in to a file. |
:doc <packageName> <functionName> | Display the documentation of given package, also function documentation if provided. |
As we understand some meta-commands, Now lets start with actual usage.
Let's type something in golang prompt and see how it behaves
go>>12312321
12312321
go>>2+3
5
go>>3-2
1
go>>5*4
20
go>>var i int
go>>i
0
go>>j:=12
go>>j
12
go>>for i:=0; i<10; i++ {
fmt.Println("Hi: ",i)
}
go>>:r
Hi: 0
Hi: 1
Hi: 2
Hi: 3
Hi: 4
Hi: 5
Hi: 6
Hi: 7
Hi: 8
Hi: 9
go>>:x
Hi: 0
Hi: 1
Hi: 2
Hi: 3
Hi: 4
Hi: 5
Hi: 6
Hi: 7
Hi: 8
Hi: 9
go>>
Hey... its working. Now let's try some errors:
go>>i=0
go>>ewedwdw
# command-line-arguments
tmp/gointerpreter/test.go:8:2: undefined: i
tmp/gointerpreter/test.go:9:14: undefined: ewedwdw
go>>
At least we are getting errors. How about importing and using function of any package:
go>>import "time"go>>time.Now()
go>>time.Now();
2018-07-12 12:51:02 Local
go>>
Note: if you want to print the output in the immediate console, please use ";" after the function call like shown above.
Now we can try arrays, map and stuctures also. Let's do it:
go>>intarr := []int{1,2,3,4,5}
go>>intarr
[1 2 3 4 5]
go>>stringarr := []string{"hello","world"}
go>>stringarr[hello world]
go>>
go>>:pp
prettyprinter enabled successfully.
go>>intarr
[]int{
1,
2,
3,
4,
5,
}
go>>stringarr
[]string{
"hello",
"world",
}
go>>
...
...
go>>mymap := make(map[string]string)
go>>mymap
map[]
go>>mymap["hello"]="world"
go>>mymap["world"]="hello"
go>>mymap
map[hello:world world:hello]
go>>
...
...
go>>type mystruct struct {
i int
s string
}
go>>var obj mystruct
go>>obj
{0 }
go>>obj2 := mystruct{1,"hello"}
go>>obj2
{1 hello}
go>>obj.i
0
go>>obj2.i
1
go>>obj2.s
hello
go>>obj.s="world"
go>>obj.s
world
go>>
After Enableing pp / pretty printer, output looks more pretty. Isn't It.. Try Yourself and see ( for other two examples map and struct ) :)
Evaluating gointerpreter's other programming constructs
I have created some animations in gif format to explain other usage of gointerpreter. For example check out below animations:
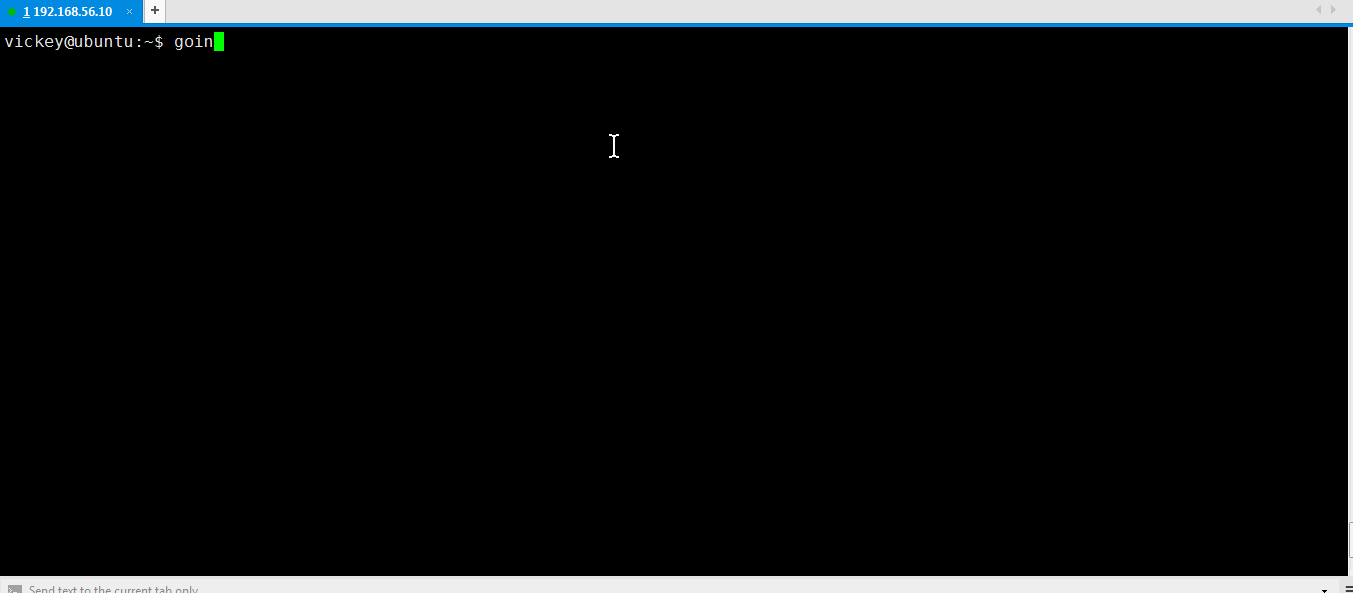
Go-interpreter also lets you use go doc to see the documentation of different packages and functions. Go interpreter is dummy REPL which internally renders and runs the files to give a REPL like feeling. so one can edit the inline codes using inbuilt editors like vim. please use ":e" command to do the same.
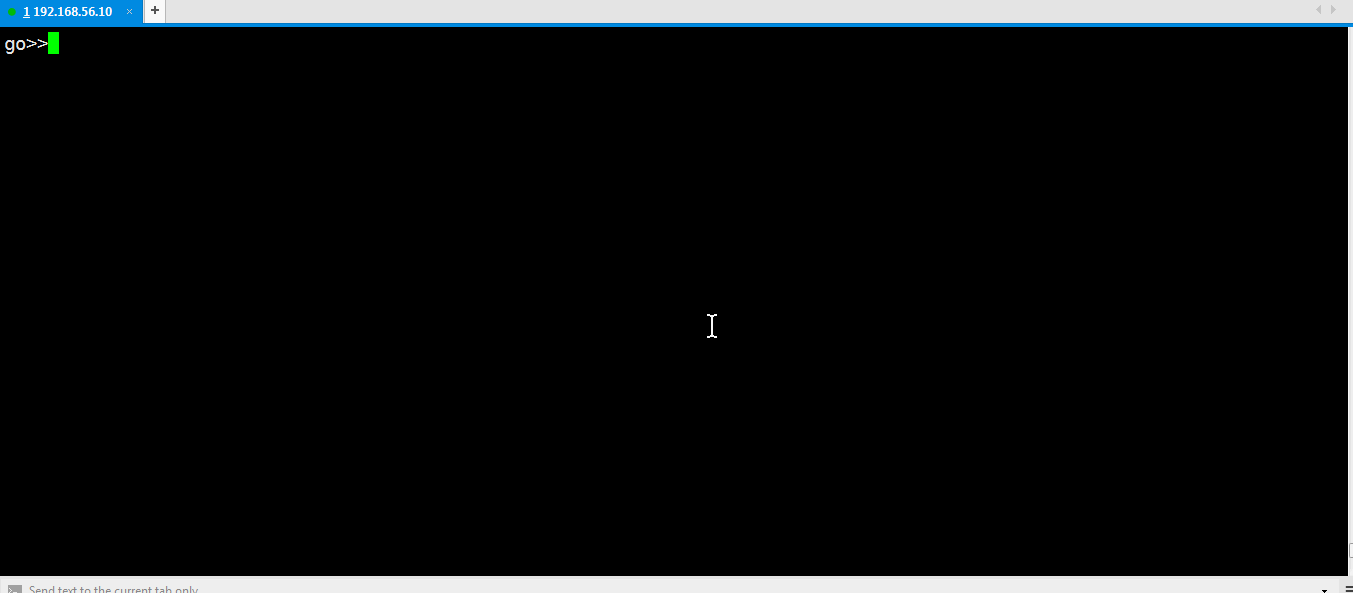
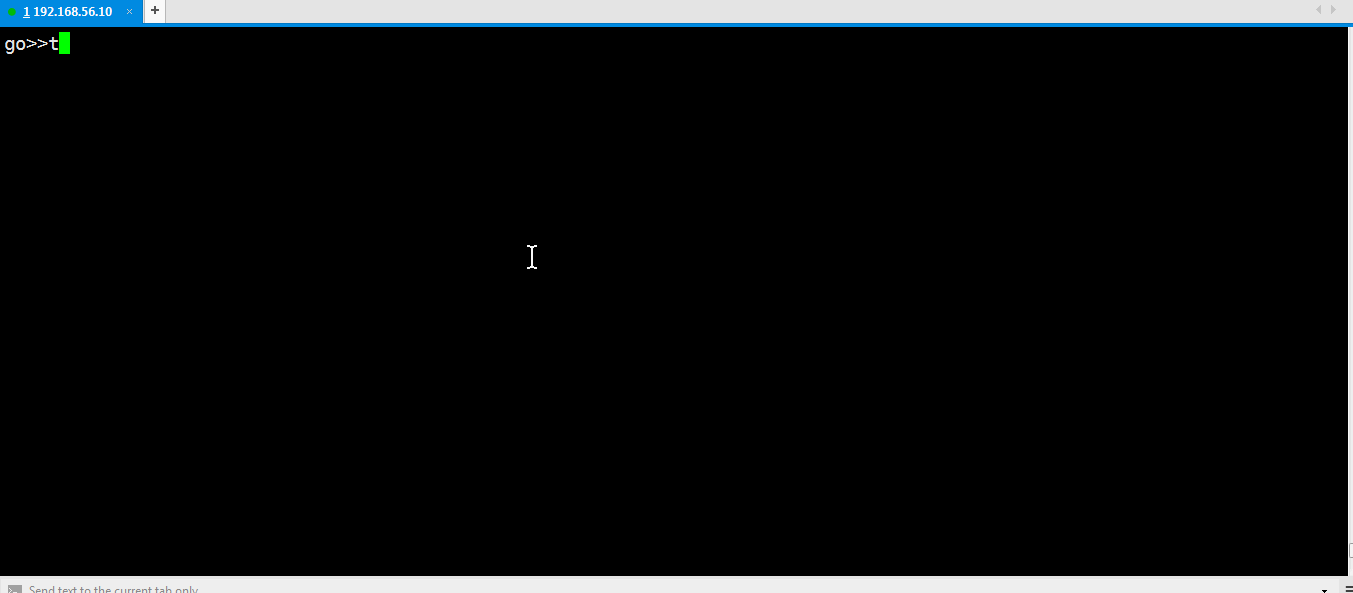
You can also use packages like encoding/json and other inbuilt packages.
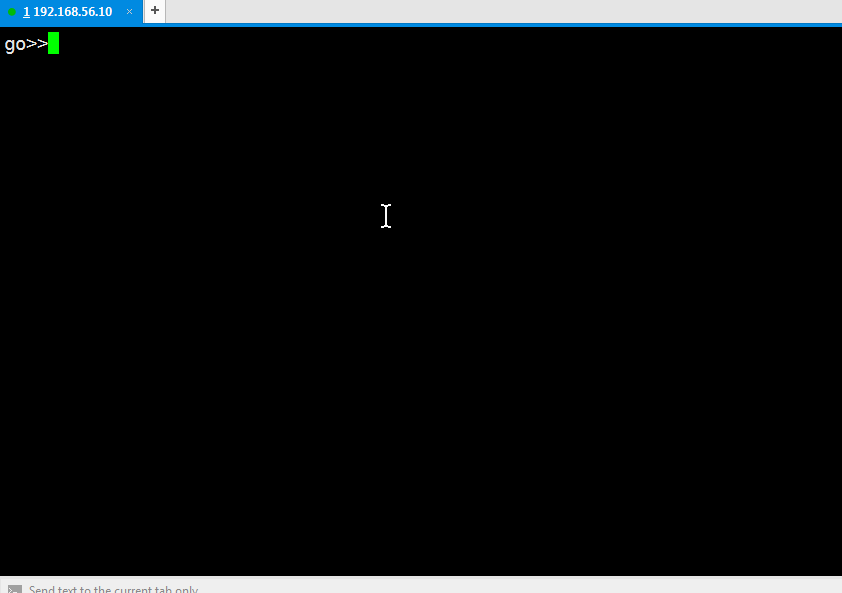
Conclusion
Woww.. we just saw that we are capable of checking and testing golang statements and constructs quickly without writing whole code. Even if it is not a full-featured REPL still, it's worth trying when learning the language.
References
Further information on golang and gointerpreter can be found in the links below: